Python Basics
Using the REPL
Let's try out a few commands in the REPL.
First, a mathematical sum to give you a flavour of what we can achieve quickly with programming. Let's find out the square of each number from 0 to 99.
For this first example, I'll use a more complex line of code so that it can be entered in a single line, though there are much simpler ways of achieving this in two or three lines rather than one:
>>> print([num * num for num in range(100)])
It should spit out the numbers pretty quick. Try playing with the number in "range", and if you want the cubes, add another asterisk to the start so it reads:
>>> print([num ** num for num in range(100)]
This code could also be represented by the following. Note the use of indentation to signal to the code - this is a key feature of python:
>>> for num in range(100):       print(num * num)
If you try entering this line by line, you'll see the REPL does not 'crunch' the line when it expects an indented line next. To add the indent, use a tab or 4 spaces (the choice is yours, but you must be consistent). Often, the text editor or REPL will add these for you, but not always.
Another quirk of the style change you may have noticed is, while the 'one-liner' printed the entire set of answers, the two-line example prints each answer on to a new line. That is, in example one we getting a list of the squares of 0 - 99 and then printing it once, while in the second we are printing the square for each of 0 - 99.
That's all the python we'll be learning for now. I encourage you to use the internet to learn more! The snippets on this site can all just be pasted directly, but it'll be a heck of a lot easier, and more customizable, the more python you know.
To quit the REPL enter quit()
to go back to the bash terminal:
>>> quit()
Writing Scripts
Entering code line by line is fun, but to save us having to do it each time we write and run scripts, which are simply files with multiple lines of code that you can run in a one-er. 'Script' is a term that is usually used pretty loosely, but basically means code which is designed to automate commands that could be entered manually. Python is good for a lot of different use cases, but especially writing scripts.
A python script is made by simply creating a file with a '.py' extension (instead of for example '.doc' or '.gif'). You can create these files in the usual way, but in the terminal you can simply enter:
$ touch filename.py
Open this up in a text editor and voila! You are now writing a python script. If you are using an editor especially for code, you might see colour coding (a.k.a. "syntax highlighting") when you enter valid python.
An even better way when starting out is to use the program named IDLE that should come with python.
When you launch it, you'll open up a python REPL, but if you press ctrl-n
to open a new file (or go to File > New in the top toolbar) it'll open up a blank file to run a script in.
Let's write and run a simple script in IDLE. In a blank file, enter:
print("hey there!")
Now press f5 (or got to Run > Run Module in the toolbar) and you'll see the program run in the REPL.
The cool thing about this, is that the REPL 'remembers' what's in the python script. So if we create a script with the following:
hello = "hey there!"
then run it, by simply entering the variable name hello
the REPL will show us the value of the 'hello' variable we set in the script.
Neat. As you can imagine, using IDLE and jumping in to the REPL is a great way to test and explore any problems you are having during the course of writing a script.
Now, let's save a file and run it from our terminal. This means we can use a program to do something for us without having to open it up in a text editor. First, save the file (we'll call it 'hiya.py') by pressing CTRL-s or using the toolbar menu File > Save As. Close down the IDLE and open up a terminal. Navigate to the folder that the hiya.py file is in using the cd (change directory) command. Now simply enter:
$ python3 hiya.py
and you'll see the result.
This places the output of the program straight in to the terminal. As a result, we can write lots of programs and have them at our fingertips using the terminal to create our own, speedy and efficient ways of working. In this example, we had to be in the right folder, but as shown in the next step, programs can be set up to be accessed from anywhere.
Calling from anywhere
When using a terminal, you will find that you can only call scripts from within the folder the file is saved, unless you want to specify the folder in our terminal, for example:
$ python3 ~/home/work_stuff/scripts/example.py
We can fix this, by adding our scripts to a folder which is specified in our PATH. The PATH is basically the list of folders the computer knows to look in when we ask it to run a program.
I'm only going to show you how to do this in Linux, but you should be able to search online for the solution for your operating system.
The traditional place to store these is in a folder called 'bin' (think receptacle rather than rubbish, though it actually stands for 'binaries'), though you can add whatever folder you want to the path. For personal scripts, the tradition is to create a 'bin' folder in the user home folder (which we already know we can jump to using cd
or cd ~
). Remember this will usually be named after your username.
In your terminal, check to make sure there isn't a folder named bin in your home folder by entering ls ~
. If no folder is there then make one with:
$ mkdir ~/bin
You can omit the ~/ if you are already in your home folder.
Now, check the folders included in your PATH by entering echo $PATH
- you may see the computer already checks for a bin folder in your personal home directory by default (e.g. home/username/bin).
If you don't see it, open up your .bashrc file in a text editor. The .bashrc file will also be in your personal home folder. If not, make one with:
$ touch .bashrc
The dot at the start of the filename means it is hidden when we are navigating around the file system unless we specifically request to see hidden files, e.g. by using ls -a
Within that file, add the following:
$ export PATH=$PATH:$HOME/bin
Now our computer knows to look in that folder for programs! To ensure your programs have permission to be run, you should make them executable by entering:
$ chmod 755 example.py
Don't worry about understanding what that means right now.
For scripts consisting of a single file, such as for quick use on the command line, you can even remove the '.py' from the filename, and instead tell the computer to use python3 from within the file itself, by adding the following line to the top of your file:
#!/usr/bin/python3
The observant among you will notice that this is directing the computer to a different 'bin' folder where the computer stores python3. Anyway, now you know how to take the scripts on this website and write and run them from anywhere on your Linux operating system. Time to get stuck in!
Installing modules
One of the benefits of Python is that there are lots of programs people have already written, whether downloaded separately or that come by default as part of the language, which can be imported in and used in your own programs.
While other programming languages have similar ways of working, python's simplicity and large community mean its "modules" are considered almost supernaturally powerful.
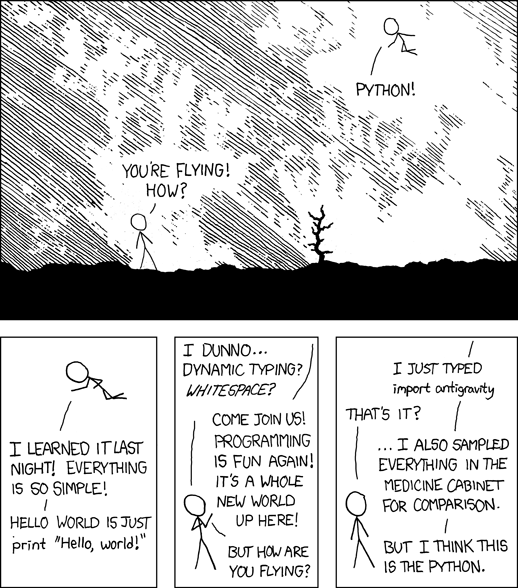
xkcd 353 (CC BY-NC 2.5)
Depending on your Linux distribution and python installation, the way you install modules on to your computer is different. The following steps explain how to to install python modules, in order of preference.
- Go to the terminal.
- Install using pip, by typing in to the terminal:
- If that didn't work, try:
- If you get an error yet again, try
sudo pip3 install docxtpl
then enter your root password - If still no dice, then check you have installed python3 correctly and you are using a bash terminal.
- In the python REPL, when you see the
>>>
prompt, import the module in to the live session by entering: - If you don't get an error after hitting return, you've installed the module correctly! Remember, when programming, output you don't ask for is usually an error.
$ pip3 install --user docxtpl
$ pip3 install docxtpl
$ from bs4 import docxtpl
Running a Script
To run a script from the terminal, open your terminal program, for example 'template.py', go to the directory you saved template.py in using the cd
command to change directories. For example, if the folder is called "programs" and sits in your home directory:
$ cd ~/programs
Check the script is indeed in your current director by using the list command to ensure the filename is listed there.
$ ls -l
Now, run the script:
$ python3 template.py
Remember, if you have made the file executable and added the python "shebang" to the top of the file (e.g. #!/usr/bin/python3), then you only need to enter the filename in the command line without specifying python3.
Once you've done that, the program should run.