Clauses on Command
Keep a suite of your favourite contract clauses at your fingertips.
The problem
Sometimes when drafting an agreement you just want to have your favourite clauses ready to paste in. Instead of raking through terms of previous agreements you have reviewed, you can paste a selection in to a csv file for quick summoning from the terminal. It also allows you to enter a new clauses from the command line, and list all the clauses available.
Tasklist
- Install the required module
- Create our script and csv file
- Run the script with different 'flags'
1. Install the modules
We are going to install 'fuzzywuzzy' so we can search for clauses without getting the exact spelling (it does a 'fuzzy' match).
We are also going to install 'click', which will let us call the script from the command line including flags to select between listing, searching and adding clauses.
2. Create our script and CSV
In the folder you want to keep the files, create the files we need with:
$ touch clauses.csv clauses.py
to create the two empty files we need.
Paste the python script below in to clauses.py and the csv data into clauses.csv
In the CSV file, you can see we've set 3 variable fields:
- The type of clause (e.g. warranty, jurisdiction). This is what the 'fuzzy' search looks for when you use it.
- The clause itself.
- A short description (to help distinguish between two clauses of the same type)
Replace the filler examples with a few of your own favourite clauses to get started:
clauses.csv
confidentiality | A party who receives Confidential Information shall not disclose it except as permitted in this agreement or otherwise agreed between the parties hereto in writing. | mutual |
confidentiality | The Receiving Party shall not disclose the Confidential Information except as permitted in this agreement or otherwise agreed between the parties hereto in writing. | one way |
jurisdiction | This agreement is governed by the laws of England and Wales and any disputes or other matters arising in relation to this agreement shall be subject to the exclusive jurisdiction of the courts of England and Wales. | england and wales |
jurisdiction | This agreement is governed by the laws of England and Wales and any disputes or other matters arising in relation to this agreement shall be subject to the exclusive jurisdiction of the courts of England and Wales. | singapore |
warranty | The service provider warrants that it shall deliver the services with due skill and care, in accordance with prevailing industry standards and applicable law. | skill and care |
assignment | Neither party may assign or otherwise transfer any of its rights or obligations under this agreement accept as agreed in writing between the parties. | only with consent |
jurisdiction | The parties agree that the courts of Spain shall have non-exclusive jurisdiction in respect of all disputes relating to or arising from this agreement. | spain |
import os
import csv
from fuzzywuzzy import fuzz
import click
file_path = "clauses.csv"
def writeClause():
title = input("Clause type: ")
text = input("Text: ")
description = input("Description: ")
with open(file_path, "a") as clause_file:
clause_writer = csv.writer(clause_file)
clause_writer.writerow([title, text, description])
def findClauses():
with open(file_path, "r") as clause_file:
reader = csv.reader(clause_file)
clause_list = [i for i in reader]
return clause_list
@click.command()
@click.option('-s')
@click.option('-l', is_flag=True)
@click.option('-n', is_flag=True)
def grabClause(s, l, n):
reader = findClauses()
if n:
writeClause()
elif l:
for index, i in enumerate(reader):
print("{}. {} - {}".format(index, i[0], i[2]))
selected_clause = input("Select: ")
try:
clause_index = int(selected_clause)
print("{}".format(reader[clause_index][1]))
except ValueError:
print("Use a number")
else:
search = findClauses()
for i in search:
if fuzz.ratio(s, i[0]) > 80:
print("{} - {}{}".format(i[0], i[2], i[1]))
if __name__ == '__main__':
grabClause()
3. Use the Flags
Using the -s flag
When we use the -s
flag we must follow it up with the term we are searching for, e.g.:
$ python3 clauses.py -s jurisdicton
This give us the following out - note how this works despite the small typo in 'jurisdiction'.
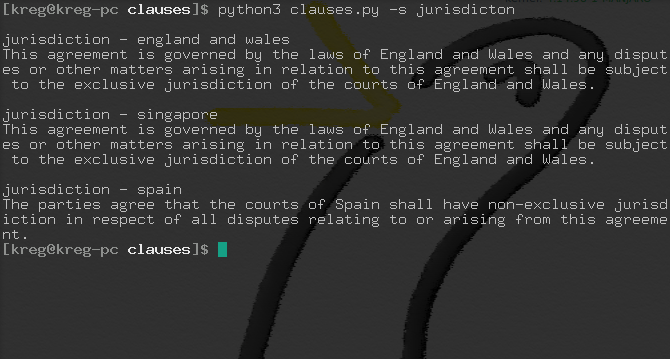
Using the -l flag
Using the -l
flag lists out all of the available clauses and their descriptions.
Then, we can select one by entering the corresponding number, as shown here:
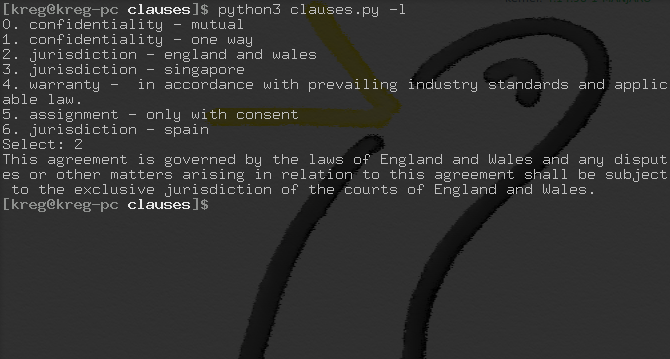
Using the -n flag
The -n
flag allows us to enter a new clause by responding to the prompts.
In the example below, we add a clause then select it from our list.
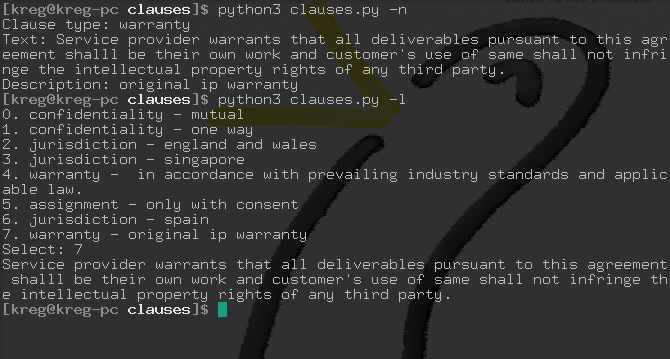