Contract Creator
Create contracts on the fly from the command line.
Automating documents is a piece of cake. We aren't talking about implementing a full contract management workflow here, just blankety-blank with a template contract, but once you can prepare your own templates a whole world of potential opens up - consider combining the below with the STF Consideration script to automate a share sale. In this example, it's literally a matter of inserting curly braces around the parts of your document which you want to replace with a variable, like a company name or a date. This method can be applied to any "docx" document you happen to have handy.
Tasklist
- Install the
docxtpl
module - Create our template in docx (as used by Microsoft Word and other word processors)
- Write the script to generate the document
- Insert the variables from within the command line
1. Install the module
The module we need to install is called "docxtpl".
If you are unsure how to install this module, check the guide at python basics.
2. Create the template
- Open up a Word Processor and select a template from one of your existing files, or create a new one.
- Replace the terms (like the company name) with a pair of curly braces ({) like in the image below. Note the highlighting is just to draw your attention - it is not required for the template to work.
- You can see we've set 4 variable fields:
- start_date
- company_number
- company_number
- term
- Save that down as 'template.docx' and our document is now complete! You can of course call the document what you want, so long as it's a docx file, but note you'll have to update the reference to 'template.html' in the script below.
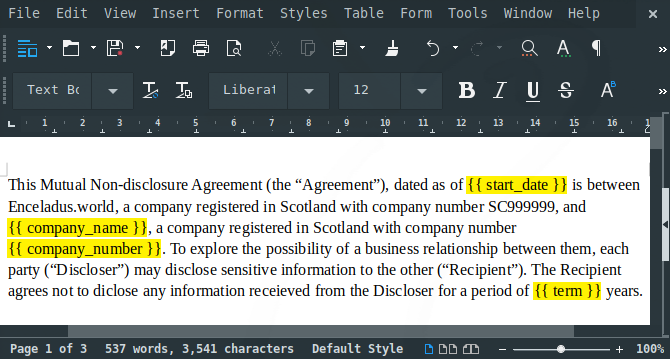
3. Write the script
This step is as simple as pasting the code below into a new python file.
- Make a new file called template.py (in the terminal you can simply enter
touch template.py
) - Open up your favourite editor, and enter the following program:
from docxtpl import DocxTemplate
from datetime import datetime
company_name = "PLEASE COMPLETE"
company_number = "PLEASE COMPLETE"
start_date = datetime.now()
start_date = start_date.strftime("%d %B %Y")
term = "5"
print("Enter values or leave blank for defaults")
name_check = input("Counterparty name: ")
num_check = input("Counterparty number: ")
date_check = input("Start Date: ")
term_check = input("Term (yrs): ")
if not name_check:
pass
else:
company_name = name_check
if not num_check:
pass
else:
company_number = num_check
if not date_check:
pass
else:
start_date = date_check
if not term_check:
pass
else:
term = term_check
doc = DocxTemplate("template.docx")
context = { 'company_name' : company_name,
'company_number' : company_number,
'start_date' : start_date,
'term' : term }
doc.render(context)
time = datetime.now()
stamp = time.strftime("%d_%b_%Y_%H%M")
file_name = "doc_{}.docx".format(stamp)
doc.save(file_name)
4. Create the document
- Open your terminal, and go to the directory you saved template.py in. Use the
cd
command to change directories. - Check the script is indeed in your current director by entering
ls -l
and ensuring the filename is listed there. - Now, run the script by entering
template.py
into the command line. - You'll be presented with a series options, which you should complete as you are asked. They all have default values built in, so leave it blank if you don't have the details to hand.
- As you can see, we've left the term and start date blank. The default value for the start date is the day you create the document.
- Once you've done that, the program should end. Check the folder it is in, and you'll see your completed template!
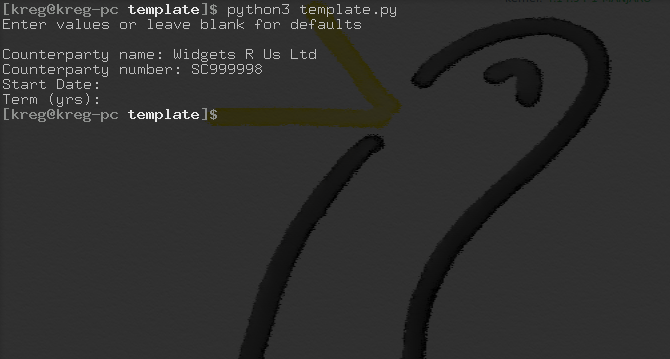
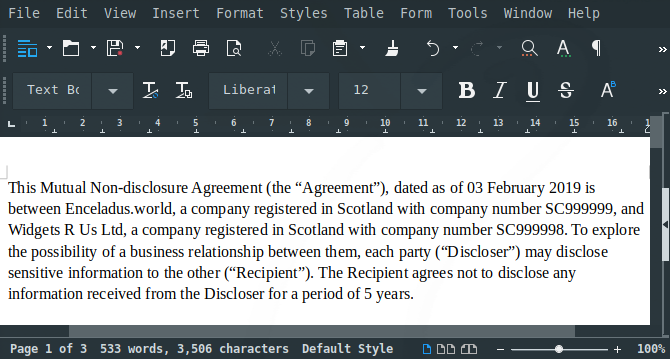