Filing
Automatically file documents.
The idea behind this script is to have a folder you can drop pdf files into. When you are ready, you can run the script and enter the necessary details to dispatch these files to their homes somewhere else.
Tasklist
- Create the folders
- Write the script
- Run the script
1. Create the folders
The first step is to create some folders to put the files in. In your case, you might want to instead point the script at existing files. In this example, we are making a file for Supplier contracts, Customer contract, Employment contracts and NDAs. We can create them all in one go like so:
$ touch supplier customer employment ndas
We've now created the folders - check it out using the ls
command if you like!
2. Write the script
In this script, if you want to make changes you need to update the pairs of values in the nested list named 'files' - the first value being the description the script will use, and the second the path to that folder on your computer. Otherwise, to work with the folder structure above, just use the below script as is.
Create a script called filer.py and paste in the following:
import os
import shutil
from datetime import datetime
def moveFile(name, target):
print("{} > {}".format(name, target))
shutil.move(name, target)
def fileDoc(filename):
files = [["Customer contracts", "./customer"],
["Supplier contracts", "./supplier"],
["NDAs", "./ndas"],
["Employment contracts", "./employment"]]
print(filename)
date = datetime.now()
date = date.strftime("%b%y")
name = filename.replace(".pdf", "")
newname = input("Rename: ")
if newname == "q":
quit()
if not newname:
name = filename
else:
name = newname
name = name + "_" + "####" + "_" + date + ".pdf"
print(name)
shutil.move(filename, name)
for index, i in enumerate(files):
print("{}. {}".format(index, i[0]))
file_type = input("File: ")
if file_type == "q":
quit()
try:
file_type = int(file_type)
except ValueError:
print("Enter a number!")
dest = files[file_type][1]
rename = files[file_type][0]
file_type = rename.split(" ")[0]
newname = name.replace("####", file_type)
shutil.move(name, newname)
moveFile(newname, dest)
for i in os.listdir('.'):
if i.endswith(".pdf"):
fileDoc(i)
We are now ready to test it out! Drag some pdf files in to the folder that contains our four new filing folders.
A quick way to do this for testing purposes is to copy and paste the same file a few times and give them different names. Here's what it looks like when I run the ls
command, though of course yours might look different:
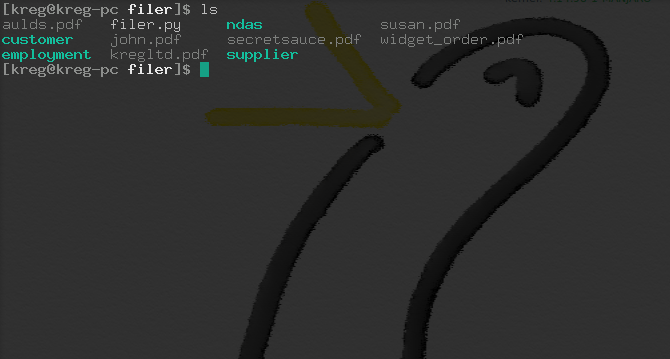
3. Run the script
Now that we have our folders made, script written, and dummy files added, we're ready to test the script.
Within the folder that contains the pdfs and the folders, enter:
$ python3 filer.py
You'll see the name of the first pdf pop up, and it will ask you to rename it. Type in the new name, or leave it blank to retain the existing name. The script will automatically append the document type, the month and year, and '.pdf' to the filename, e.g. newcontract_employment_Mar19.pdf
You'll then see your choice of folders with a corresponding number - enter the corresponding number to send the file to that destination.
You can enter 'q' at any question to quit the program. Files already filed or renamed before then will stay filed and renamed.
Once I've finished answering the questions, the files are all neatly stowed away, as evidenced by running the "tree" command to output the current folders and contents:
$ tree
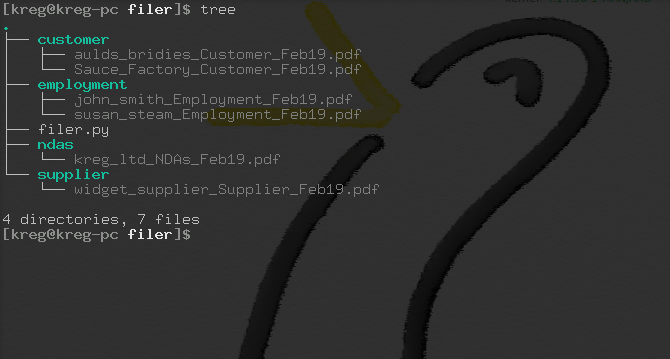
Ta da! Although this script only works with pdfs, it can easily be changed to any other filetypes - why not see if you can figure out how to edit the script to pick up other kinds of files?